b_asic.core_operations
¶
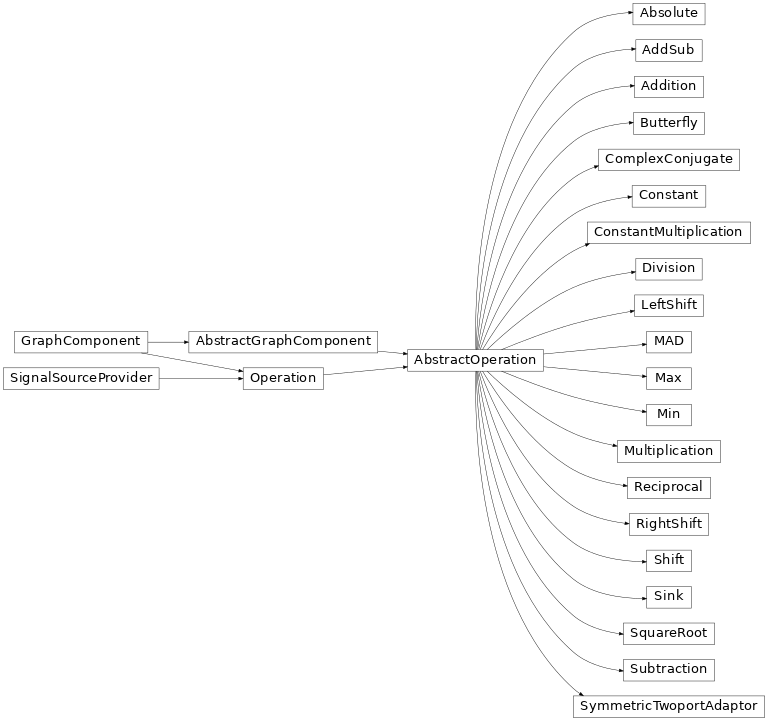
B-ASIC Core Operations Module.
Contains some of the most commonly used mathematical operations.
- class b_asic.core_operations.Absolute(src0: SignalSourceProvider | None = None, name: str = '', latency: int | None = None, latency_offsets: Dict[str, int] | None = None, execution_time: int | None = None)¶
Bases:
AbstractOperation
Absolute value operation.
Gives the absolute value of its input.
\[y = |x|\]- Parameters:
- src0
SignalSourceProvider
, optional The signal to compute the absolute value of.
- nameName, optional
Operation name.
- latencyint, optional
Operation latency (delay from input to output in time units).
- latency_offsetsdict[str, int], optional
Used if input arrives later than when the operator starts, e.g.,
{"in0": 0
which corresponds to src0 arriving one time unit after the operator starts. If not provided and latency is provided, set to zero.- execution_timeint, optional
Operation execution time (time units before operator can be reused).
- src0
- evaluate(a)¶
Evaluate the operation and generate a list of output values.
- Parameters:
- *inputs
List of input values.
- classmethod type_name() TypeName ¶
Get the type name of this graph component.
- class b_asic.core_operations.AddSub(is_add: bool = True, src0: SignalSourceProvider | None = None, src1: SignalSourceProvider | None = None, name: str = '', latency: int | None = None, latency_offsets: Dict[str, int] | None = None, execution_time: int | None = None)¶
Bases:
AbstractOperation
Two-input addition or subtraction operation.
Gives the result of adding or subtracting two inputs.
\[\begin{split}y = \begin{cases} x_0 + x_1,& \text{is_add} = \text{True}\\ x_0 - x_1,& \text{is_add} = \text{False} \end{cases}\end{split}\]This is used to later map additions and subtractions to the same operator.
- Parameters:
- is_addbool, default: True
If True, the operation is an addition, if False, a subtraction.
- src0, src1SignalSourceProvider, optional
The two signals to add or subtract.
- nameName, optional
Operation name.
- latencyint, optional
Operation latency (delay from input to output in time units).
- latency_offsetsdict[str, int], optional
Used if inputs have different arrival times, e.g.,
{"in0": 0, "in1": 1}
which corresponds to src1 arriving one time unit later than src0. If not provided and latency is provided, set to zero if not explicitly provided. So the previous example can be written as{"in1": 1}
only.- execution_timeint, optional
Operation execution time (time units before operator can be reused).
See also
- evaluate(a, b)¶
Evaluate the operation and generate a list of output values.
- Parameters:
- *inputs
List of input values.
- is_linear = True¶
- property is_swappable: bool¶
Return True if the inputs (and outputs) to the operation can be swapped.
Swapping require that the operation retains the same function, but it is allowed to modify values to do so.
- classmethod type_name() TypeName ¶
Get the type name of this graph component.
- class b_asic.core_operations.Addition(src0: SignalSourceProvider | None = None, src1: SignalSourceProvider | None = None, name: str = '', latency: int | None = None, latency_offsets: Dict[str, int] | None = None, execution_time: int | None = None)¶
Bases:
AbstractOperation
Binary addition operation.
Gives the result of adding two inputs.
\[y = x_0 + x_1\]- Parameters:
- src0, src1SignalSourceProvider, optional
The two signals to add.
- nameName, optional
Operation name.
- latencyint, optional
Operation latency (delay from input to output in time units).
- latency_offsetsdict[str, int], optional
Used if inputs have different arrival times, e.g.,
{"in0": 0, "in1": 1}
which corresponds to src1 arriving one time unit later than src0. If not provided and latency is provided, set to zero if not explicitly provided. So the previous example can be written as{"in1": 1}
only.- execution_timeint, optional
Operation execution time (time units before operator can be reused).
See also
- evaluate(a, b)¶
Evaluate the operation and generate a list of output values.
- Parameters:
- *inputs
List of input values.
- is_linear = True¶
- is_swappable = True¶
- classmethod type_name() TypeName ¶
Get the type name of this graph component.
- class b_asic.core_operations.Butterfly(src0: SignalSourceProvider | None = None, src1: SignalSourceProvider | None = None, name: str = '', latency: int | None = None, latency_offsets: Dict[str, int] | None = None, execution_time: int | None = None)¶
Bases:
AbstractOperation
Radix-2 Butterfly operation for FFTs.
Gives the result of adding its two inputs, as well as the result of subtracting the second input from the first one. This corresponds to a 2-point DFT.
\[\begin{split}\begin{eqnarray} y_0 & = & x_0 + x_1\\ y_1 & = & x_0 - x_1 \end{eqnarray}\end{split}\]- Parameters:
- src0, src1SignalSourceProvider, optional
The two signals to compute the 2-point DFT of.
- nameName, optional
Operation name.
- latencyint, optional
Operation latency (delay from input to output in time units).
- latency_offsetsdict[str, int], optional
Used if inputs have different arrival times or if the inputs should arrive after the operator has stared. For example,
{"in0": 0, "in1": 1}
which corresponds to src1 arriving one time unit later than src0 and one time unit later than the operator starts. If not provided and latency is provided, set to zero. Hence, the previous example can be written as{"in1": 1}
only.- execution_timeint, optional
Operation execution time (time units before operator can be reused).
- evaluate(a, b)¶
Evaluate the operation and generate a list of output values.
- Parameters:
- *inputs
List of input values.
- is_linear = True¶
- classmethod type_name() TypeName ¶
Get the type name of this graph component.
- class b_asic.core_operations.ComplexConjugate(src0: SignalSourceProvider | None = None, name: str = '', latency: int | None = None, latency_offsets: Dict[str, int] | None = None, execution_time: int | None = None)¶
Bases:
AbstractOperation
Complex conjugate operation.
Gives the complex conjugate of its input.
\[y = x^*\]- Parameters:
- src0
SignalSourceProvider
, optional The signal to compute the complex conjugate of.
- nameName, optional
Operation name.
- latencyint, optional
Operation latency (delay from input to output in time units).
- latency_offsetsdict[str, int], optional
Used if input arrives later than when the operator starts, e.g.,
{"in0": 0
which corresponds to src0 arriving one time unit after the operator starts. If not provided and latency is provided, set to zero.- execution_timeint, optional
Operation execution time (time units before operator can be reused).
- src0
- evaluate(a)¶
Evaluate the operation and generate a list of output values.
- Parameters:
- *inputs
List of input values.
- classmethod type_name() TypeName ¶
Get the type name of this graph component.
- class b_asic.core_operations.Constant(value: int | float | complex = 0, name: str = '')¶
Bases:
AbstractOperation
Constant value operation.
Gives a specified value that remains constant for every iteration.
\[y = \text{value}\]- Parameters:
- valueNumber, default: 0
The constant value.
- nameName, optional
Operation name.
- evaluate()¶
Evaluate the operation and generate a list of output values.
- Parameters:
- *inputs
List of input values.
- is_constant = True¶
- is_linear = True¶
- property latency: int¶
Get the latency of the operation.
This is the longest time it takes from one of the input ports to one of the output ports.
- classmethod type_name() TypeName ¶
Get the type name of this graph component.
- class b_asic.core_operations.ConstantMultiplication(value: int | float | complex = 0, src0: SignalSourceProvider | None = None, name: str = '', latency: int | None = None, latency_offsets: Dict[str, int] | None = None, execution_time: int | None = None)¶
Bases:
AbstractOperation
Constant multiplication operation.
Gives the result of multiplying its input by a specified value.
\[y = x_0 \times \text{value}\]- Parameters:
- valueint
Value to multiply with.
- src0
SignalSourceProvider
, optional The signal to multiply.
- nameName, optional
Operation name.
- latencyint, optional
Operation latency (delay from input to output in time units).
- latency_offsetsdict[str, int], optional
Used if input arrives later than when the operator starts, e.g.,
{"in0": 0
which corresponds to src0 arriving one time unit after the operator starts. If not provided and latency is provided, set to zero.- execution_timeint, optional
Operation execution time (time units before operator can be reused).
See also
- evaluate(a)¶
Evaluate the operation and generate a list of output values.
- Parameters:
- *inputs
List of input values.
- is_linear = True¶
- classmethod type_name() TypeName ¶
Get the type name of this graph component.
- class b_asic.core_operations.Division(src0: SignalSourceProvider | None = None, src1: SignalSourceProvider | None = None, name: str = '', latency: int | None = None, latency_offsets: Dict[str, int] | None = None, execution_time: int | None = None)¶
Bases:
AbstractOperation
Binary division operation.
Gives the result of dividing the first input by the second one.
\[y = \frac{x_0}{x_1}\]- Parameters:
- src0, src1SignalSourceProvider, optional
The two signals to divide.
- nameName, optional
Operation name.
- latencyint, optional
Operation latency (delay from input to output in time units).
- latency_offsetsdict[str, int], optional
Used if inputs have different arrival times or if the inputs should arrive after the operator has stared. For example,
{"in0": 0, "in1": 1}
which corresponds to src1 arriving one time unit later than src0 and one time unit later than the operator starts. If not provided and latency is provided, set to zero. Hence, the previous example can be written as{"in1": 1}
only.- execution_timeint, optional
Operation execution time (time units before operator can be reused).
See also
- evaluate(a, b)¶
Evaluate the operation and generate a list of output values.
- Parameters:
- *inputs
List of input values.
- classmethod type_name() TypeName ¶
Get the type name of this graph component.
- class b_asic.core_operations.LeftShift(value: int = 0, src0: SignalSourceProvider | None = None, name: str = '', latency: int | None = None, latency_offsets: Dict[str, int] | None = None, execution_time: int | None = None)¶
Bases:
AbstractOperation
Arithmetic left-shift operation.
Shifts the input to the left assuming a fixed-point representation, so a multiplication by a power of two.
\[y = x \ll \text{value} = 2^{\text{value}}x \text{ where value} \geq 0\]- Parameters:
- valueint
Number of bits to shift left.
- src0
SignalSourceProvider
, optional The signal to shift left.
- nameName, optional
Operation name.
- latencyint, optional
Operation latency (delay from input to output in time units).
- latency_offsetsdict[str, int], optional
Used if input arrives later than when the operator starts, e.g.,
{"in0": 0
which corresponds to src0 arriving one time unit after the operator starts. If not provided and latency is provided, set to zero.- execution_timeint, optional
Operation execution time (time units before operator can be reused).
See also
- evaluate(a)¶
Evaluate the operation and generate a list of output values.
- Parameters:
- *inputs
List of input values.
- is_linear = True¶
- classmethod type_name() TypeName ¶
Get the type name of this graph component.
- class b_asic.core_operations.MAD(src0: SignalSourceProvider | None = None, src1: SignalSourceProvider | None = None, src2: SignalSourceProvider | None = None, name: str = '', latency: int | None = None, latency_offsets: Dict[str, int] | None = None, execution_time: int | None = None)¶
Bases:
AbstractOperation
Multiply-add operation.
Gives the result of multiplying the first input by the second input and then adding the third input.
\[y = x_0 \times x_1 + x_2\]- Parameters:
- src0, src1, src2SignalSourceProvider, optional
The three signals to determine the multiply-add operation of.
- nameName, optional
Operation name.
- latencyint, optional
Operation latency (delay from input to output in time units).
- latency_offsetsdict[str, int], optional
Used if inputs have different arrival times or if the inputs should arrive after the operator has stared. For example,
{"in0": 0, "in1": 0, "in2": 2}
which corresponds to src2, i.e., the term to be added, arriving two time units later than src0 and src1. If not provided and latency is provided, set to zero. Hence, the previous example can be written as{"in1": 1}
only.- execution_timeint, optional
Operation execution time (time units before operator can be reused).
See also
- evaluate(a, b, c)¶
Evaluate the operation and generate a list of output values.
- Parameters:
- *inputs
List of input values.
- is_swappable = True¶
- swap_io() None ¶
Swap inputs (and outputs) of operation.
Errors if
is_swappable()
is False.
- classmethod type_name() TypeName ¶
Get the type name of this graph component.
- class b_asic.core_operations.Max(src0: SignalSourceProvider | None = None, src1: SignalSourceProvider | None = None, name: str = '', latency: int | None = None, latency_offsets: Dict[str, int] | None = None, execution_time: int | None = None)¶
Bases:
AbstractOperation
Binary max operation.
Gives the maximum value of two inputs.
\[y = \max\{x_0 , x_1\}\]Note
Only real-valued numbers are supported.
- Parameters:
- src0, src1SignalSourceProvider, optional
The two signals to determine the min of.
- nameName, optional
Operation name.
- latencyint, optional
Operation latency (delay from input to output in time units).
- latency_offsetsdict[str, int], optional
Used if inputs have different arrival times or if the inputs should arrive after the operator has stared. For example,
{"in0": 0, "in1": 1}
which corresponds to src1 arriving one time unit later than src0 and one time unit later than the operator starts. If not provided and latency is provided, set to zero. Hence, the previous example can be written as{"in1": 1}
only.- execution_timeint, optional
Operation execution time (time units before operator can be reused).
See also
- evaluate(a, b)¶
Evaluate the operation and generate a list of output values.
- Parameters:
- *inputs
List of input values.
- is_swappable = True¶
- classmethod type_name() TypeName ¶
Get the type name of this graph component.
- class b_asic.core_operations.Min(src0: SignalSourceProvider | None = None, src1: SignalSourceProvider | None = None, name: str = '', latency: int | None = None, latency_offsets: Dict[str, int] | None = None, execution_time: int | None = None)¶
Bases:
AbstractOperation
Binary min operation.
Gives the minimum value of two inputs.
\[y = \min\{x_0 , x_1\}\]Note
Only real-valued numbers are supported.
- Parameters:
- src0, src1SignalSourceProvider, optional
The two signals to determine the min of.
- nameName, optional
Operation name.
- latencyint, optional
Operation latency (delay from input to output in time units).
- latency_offsetsdict[str, int], optional
Used if inputs have different arrival times or if the inputs should arrive after the operator has stared. For example,
{"in0": 0, "in1": 1}
which corresponds to src1 arriving one time unit later than src0 and one time unit later than the operator starts. If not provided and latency is provided, set to zero. Hence, the previous example can be written as{"in1": 1}
only.- execution_timeint, optional
Operation execution time (time units before operator can be reused).
See also
- evaluate(a, b)¶
Evaluate the operation and generate a list of output values.
- Parameters:
- *inputs
List of input values.
- is_swappable = True¶
- classmethod type_name() TypeName ¶
Get the type name of this graph component.
- class b_asic.core_operations.Multiplication(src0: SignalSourceProvider | None = None, src1: SignalSourceProvider | None = None, name: str = '', latency: int | None = None, latency_offsets: Dict[str, int] | None = None, execution_time: int | None = None)¶
Bases:
AbstractOperation
Binary multiplication operation.
Gives the result of multiplying two inputs.
\[y = x_0 \times x_1\]- Parameters:
- src0, src1SignalSourceProvider, optional
The two signals to multiply.
- nameName, optional
Operation name.
- latencyint, optional
Operation latency (delay from input to output in time units).
- latency_offsetsdict[str, int], optional
Used if inputs have different arrival times or if the inputs should arrive after the operator has stared. For example,
{"in0": 0, "in1": 1}
which corresponds to src1 arriving one time unit later than src0 and one time unit later than the operator starts. If not provided and latency is provided, set to zero. Hence, the previous example can be written as{"in1": 1}
only.- execution_timeint, optional
Operation execution time (time units before operator can be reused).
See also
- evaluate(a, b)¶
Evaluate the operation and generate a list of output values.
- Parameters:
- *inputs
List of input values.
- is_swappable = True¶
- classmethod type_name() TypeName ¶
Get the type name of this graph component.
- class b_asic.core_operations.Reciprocal(src0: SignalSourceProvider | None = None, name: str = '', latency: int | None = None, latency_offsets: Dict[str, int] | None = None, execution_time: int | None = None)¶
Bases:
AbstractOperation
Reciprocal operation.
Gives the reciprocal of its input.
\[y = \frac{1}{x}\]- Parameters:
- src0
SignalSourceProvider
, optional The signal to compute the reciprocal of.
- nameName, optional
Operation name.
- latencyint, optional
Operation latency (delay from input to output in time units).
- latency_offsetsdict[str, int], optional
Used if input arrives later than when the operator starts, e.g.,
{"in0": 0
which corresponds to src0 arriving one time unit after the operator starts. If not provided and latency is provided, set to zero.- execution_timeint, optional
Operation execution time (time units before operator can be reused).
- src0
See also
- evaluate(a)¶
Evaluate the operation and generate a list of output values.
- Parameters:
- *inputs
List of input values.
- classmethod type_name() TypeName ¶
Get the type name of this graph component.
- class b_asic.core_operations.RightShift(value: int = 0, src0: SignalSourceProvider | None = None, name: str = '', latency: int | None = None, latency_offsets: Dict[str, int] | None = None, execution_time: int | None = None)¶
Bases:
AbstractOperation
Arithmetic right-shift operation.
Shifts the input to the right assuming a fixed-point representation, so a multiplication by a power of two.
\[y = x \gg \text{value} = 2^{-\text{value}}x \text{ where value} \geq 0\]- Parameters:
- valueint
Number of bits to shift right.
- src0
SignalSourceProvider
, optional The signal to shift right.
- nameName, optional
Operation name.
- latencyint, optional
Operation latency (delay from input to output in time units).
- latency_offsetsdict[str, int], optional
Used if input arrives later than when the operator starts, e.g.,
{"in0": 0
which corresponds to src0 arriving one time unit after the operator starts. If not provided and latency is provided, set to zero.- execution_timeint, optional
Operation execution time (time units before operator can be reused).
- evaluate(a)¶
Evaluate the operation and generate a list of output values.
- Parameters:
- *inputs
List of input values.
- is_linear = True¶
- classmethod type_name() TypeName ¶
Get the type name of this graph component.
- class b_asic.core_operations.Shift(value: int = 0, src0: SignalSourceProvider | None = None, name: str = '', latency: int | None = None, latency_offsets: Dict[str, int] | None = None, execution_time: int | None = None)¶
Bases:
AbstractOperation
Arithmetic shift operation.
Shifts the input to the left or right assuming a fixed-point representation, so a multiplication by a power of two. By definition a positive value is a shift to the left.
\[y = x \ll \text{value} = 2^{\text{value}}x\]- Parameters:
- valueint
Number of bits to shift. Positive value shifts to the left.
- src0
SignalSourceProvider
, optional The signal to shift.
- nameName, optional
Operation name.
- latencyint, optional
Operation latency (delay from input to output in time units).
- latency_offsetsdict[str, int], optional
Used if input arrives later than when the operator starts, e.g.,
{"in0": 0
which corresponds to src0 arriving one time unit after the operator starts. If not provided and latency is provided, set to zero.- execution_timeint, optional
Operation execution time (time units before operator can be reused).
See also
- evaluate(a)¶
Evaluate the operation and generate a list of output values.
- Parameters:
- *inputs
List of input values.
- is_linear = True¶
- classmethod type_name() TypeName ¶
Get the type name of this graph component.
- class b_asic.core_operations.Sink(name: str = '')¶
Bases:
AbstractOperation
Sink operation.
Used for ignoring the output from another operation to avoid dangling output nodes.
- Parameters:
- nameName, optional
Operation name.
- evaluate()¶
Evaluate the operation and generate a list of output values.
- Parameters:
- *inputs
List of input values.
- is_linear = True¶
- property latency: int¶
Get the latency of the operation.
This is the longest time it takes from one of the input ports to one of the output ports.
- classmethod type_name() TypeName ¶
Get the type name of this graph component.
- class b_asic.core_operations.SquareRoot(src0: SignalSourceProvider | None = None, name: str = '', latency: int | None = None, latency_offsets: Dict[str, int] | None = None, execution_time: int | None = None)¶
Bases:
AbstractOperation
Square root operation.
Gives the square root of its input.
\[y = \sqrt{x}\]- Parameters:
- src0
SignalSourceProvider
, optional The signal to compute the square root of.
- nameName, optional
Operation name.
- latencyint, optional
Operation latency (delay from input to output in time units).
- latency_offsetsdict[str, int], optional
Used if input arrives later than when the operator starts, e.g.,
{"in0": 0
which corresponds to src0 arriving one time unit after the operator starts. If not provided and latency is provided, set to zero.- execution_timeint, optional
Operation execution time (time units before operator can be reused).
- src0
- evaluate(a)¶
Evaluate the operation and generate a list of output values.
- Parameters:
- *inputs
List of input values.
- classmethod type_name() TypeName ¶
Get the type name of this graph component.
- class b_asic.core_operations.Subtraction(src0: SignalSourceProvider | None = None, src1: SignalSourceProvider | None = None, name: str = '', latency: int | None = None, latency_offsets: Dict[str, int] | None = None, execution_time: int | None = None)¶
Bases:
AbstractOperation
Binary subtraction operation.
Gives the result of subtracting the second input from the first one.
\[y = x_0 - x_1\]- Parameters:
- src0, src1SignalSourceProvider, optional
The two signals to subtract.
- nameName, optional
Operation name.
- latencyint, optional
Operation latency (delay from input to output in time units).
- latency_offsetsdict[str, int], optional
Used if inputs have different arrival times, e.g.,
{"in0": 0, "in1": 1}
which corresponds to src1 arriving one time unit later than src0. If not provided and latency is provided, set to zero if not explicitly provided. So the previous example can be written as{"in1": 1}
only.- execution_timeint, optional
Operation execution time (time units before operator can be reused).
See also
- evaluate(a, b)¶
Evaluate the operation and generate a list of output values.
- Parameters:
- *inputs
List of input values.
- is_linear = True¶
- classmethod type_name() TypeName ¶
Get the type name of this graph component.
- class b_asic.core_operations.SymmetricTwoportAdaptor(value: int | float | complex = 0, src0: SignalSourceProvider | None = None, src1: SignalSourceProvider | None = None, name: str = '', latency: int | None = None, latency_offsets: Dict[str, int] | None = None, execution_time: int | None = None)¶
Bases:
AbstractOperation
Wave digital filter symmetric twoport-adaptor operation.
\[\begin{split}\begin{eqnarray} y_0 & = & x_1 + \text{value}\times\left(x_1 - x_0\right)\\ y_1 & = & x_0 + \text{value}\times\left(x_1 - x_0\right) \end{eqnarray}\end{split}\]- evaluate(a, b)¶
Evaluate the operation and generate a list of output values.
- Parameters:
- *inputs
List of input values.
- is_linear = True¶
- is_swappable = True¶
- swap_io() None ¶
Swap inputs (and outputs) of operation.
Errors if
is_swappable()
is False.
- classmethod type_name() TypeName ¶
Get the type name of this graph component.